Random number generation with OpenMP and MPI
In this parallel simulation using OpenMP and MPI, a coin is thrown many times and the number of tails is counted. The probability should be exactly 1/2.
This code is a backbone implementation of a parallelized loop using a thread-safe (reentrant) random number generator (drand48_r), uniformly distributed over the [0,1) interval, with MPI and OpenMP.
This backbone code (random_omp_mpi.c) is well-documented with comments and instructions, and can be used to perform other parallel simulations that are implemented over a loop and use random numbers.
Demo
The execution time is used to define the performance, measured in Mega trials per second. In an 8 processor computer with 16 threads, running 4 processors, each with 4 threads, and 2 billion tosses, the performance on average is about 1080 megatrials per second and takes about 1.9 seconds.
using message-passing interface (MPI) with 4 processors
processor: 2 thread: 0 seed: 25997512
processor: 2 thread: 3 seed: 1368825539
processor: 2 thread: 2 seed: 1308127545
processor: 1 thread: 0 seed: 670679397
processor: 1 thread: 2 seed: 482681226
processor: 1 thread: 1 seed: 782324012
processor: 1 thread: 3 seed: 141267155
processor: 0 thread: 0 seed: 84023690
processor: 0 thread: 3 seed: 255496263
processor: 0 thread: 1 seed: 429453065
processor: 0 thread: 2 seed: 1468860505
processor: 2 thread: 1 seed: 1923864576
processor: 3 thread: 0 seed: 232466065
processor: 3 thread: 3 seed: 1457437729
processor: 3 thread: 2 seed: 623692409
processor: 3 thread: 1 seed: 881189643
number of tosses: 2000000000
number of tails: 1000020502
probability: 0.500010251
execution time: 1.84868 s
performance: 1081.85 Mtrials/sec
The following plot, using 4 processors and 4 threads per processor, shows the well-known behavior of performance with increasing problem size,
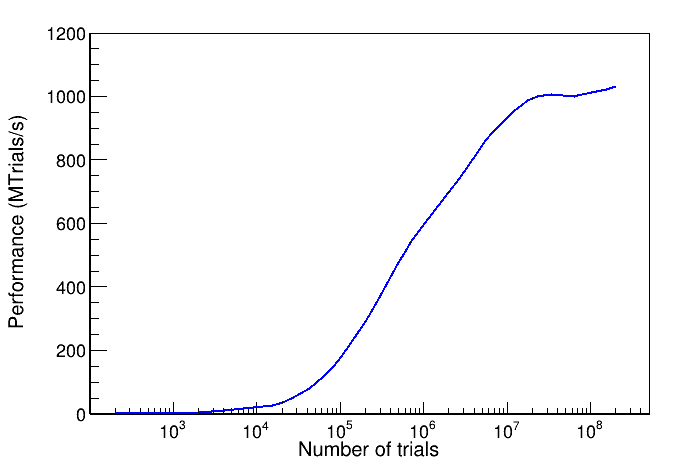
Compilation and execution
Compile the code with,
and execute with the command line,
where num_procs is the number of processors and num_tries is the number of coin tosses. To suppress the output to stderr,
Dependencies
This software has been developed and tested using the GNU GCC compiler with OpenMP support, and OpenMPI.
To determine if your GCC compiler has OpenMP support, execute the following command,
If the response is non-empty, for example,
the compiler has built-in OpenMP support. The _OPENMP preprocessor macro defines the year and month of the implemented OpenMP version, in decimal value format yyyymm.
OpenMPI is open source and part of most major Linux distributions. On Debian-based systems, OpenMPI can be installed with the command,Whichever MPI implementation is used, it is essential that both the compilation of the code and execution is made with the same implementation.
Terms of Use and License
Before buying, please read our Terms of Use and License.
List of Files
Included in this software package are the following files:
- random_omp_mpi.c (C code)
- README (documentation)
- terms.md (terms of use)
- license.md (license)